Highlight
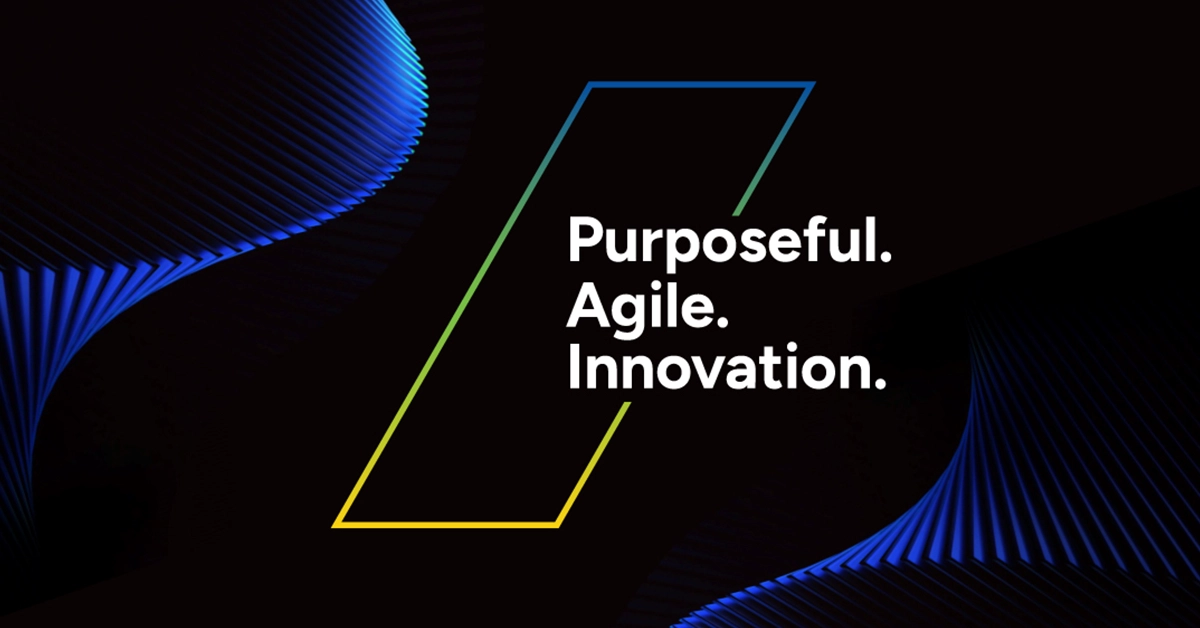
Purposeful. Agile. Innovation.
The shifting patterns of migration have a profound impact on global economic dynamics, driving the emergence of a new middle class. This transformation demands a re-evaluation of solutions and product...
The shifting patterns of migration have a profound impact on global economic dynamics, driving the emergence of a new middle class. This transformation demands a re-evaluation of solutions and product...